Imagine yourself in a situation where you need to lookup geolocation for a visitor’s IP address. You need it to tailor the content of your site for a visitor from a specific region or country for example. Using PHP to get the IP address will require you to request this info from a PHP GeoIP service like IP Geolocation, after which you’ll get back a response with everything you need.
Parameters to work with
The primary way to get geolocation information is using the call method get_geolocation($apiKey, $ip, $lang, $fields, $excludes). Using just the get_geolocation will call back all info available from the IP address, but the other parameters are here so you can augment your response depending on the specific information needed.
The first two parameters are quite self-explanatory. With $apiKey being your API key you’ll have to generate in order to use IP Geolocation, while the $ip represents the visitors IP from which the info will be pulled. The rest of them are essentially customization options for your response.
$lang is a really great IP Geolocation option that gives you a multilingual response option. While the default response language is English (en), you’ll be able to get said response in other languages if you so choose. These alternate languages include German (de), Russian (ru), Japanese (ja), French (fr), Chinese Simplified (cn), Spanish (es), Czech (cs) and Italian (it). The designation in brackets represents how you’ll label each language while writing them into the code. It’s important to note that all alternate language options are available only in premium tiers, while the free tier only has the default English as an option for responses.
$fields will enable you to choose which fields are to be included in the response. There may be times when you don’t want to get back all geolocation info, but just some of it – this is where you’ll use this command. Similarly, $excludes makes the response exclude specific fields from the whole response. Both of these parameters are used in a certain situation, while most of the time you’ll probably be using the regular, full response.
An example of getting a response displayed as a table would look like this (the key parameters are highlighted in red):
<style>
table, th, tr, td {
border: 1px solid black;
border-collapse: collapse;
}
th, td {
padding: 5px 30px;
}
</style>
<?php
$apiKey = "PUT_YOUR_API_KEY_HERE";
$ips = array("93.141.113.150", "72.229.28.185", "196.245.163.202", "6.6.6.6", "155.94.166.104");
echo "<table>";
echo "<tr>";
echo "<th>IP</th>";
echo "<th>Continent</th>";
echo "<th>Country</th>";
echo "<th>Organization</th>";
echo "<th>ISP</th>";
echo "<th>Languages</th>";
echo "<th>Is EU Member?</th>";
echo "<th>Currency</th>";
echo "<th>Timezone</th>";
echo "</tr>";
foreach ($ips as $ip) {
$location = get_geolocation($apiKey, $ip);
$decodedLocation = json_decode($location, true);
echo "<tr>";
echo "<td>".$decodedLocation['ip']."</td>";
echo "<td>".$decodedLocation['continent_name']." (".$decodedLocation['continent_code'].")</td>";
echo "<td>".$decodedLocation['country_name']." (".$decodedLocation['country_code2'].")</td>";
echo "<td>".$decodedLocation['organization']."</td>";
echo "<td>".$decodedLocation['isp']."</td>";
echo "<td>".$decodedLocation['languages']."</td>";
if($decodedLocation['is_eu'] == true) {
echo "<td>Yes</td>";
} else {
echo "<td>No</td>";
}
echo "<td>".$decodedLocation['currency']['name']."</td>";
echo "<td>".$decodedLocation['time_zone']['name']."</td>";
echo "</tr>";
}
echo "</table>";
function get_geolocation($apiKey, $ip, $lang = "en", $fields = "*", $excludes = "") {
$url = "https://api.ipgeolocation.io/ipgeo?apiKey=".$apiKey."&ip=".$ip."&lang=".$lang."&fields=".$fields."&excludes=".$excludes;
$cURL = curl_init();
curl_setopt($cURL, CURLOPT_URL, $url);
curl_setopt($cURL, CURLOPT_HTTPGET, true);
curl_setopt($cURL, CURLOPT_RETURNTRANSFER, true);
curl_setopt($cURL, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json',
'Accept: application/json'
));
return curl_exec($cURL);
}
?>
Pricing
Now that you know how requesting geolocation works, you’ll probably want to know how much it costs. IP Geolocation houses a vast array of premium tiers to cover basically all possible request volumes.
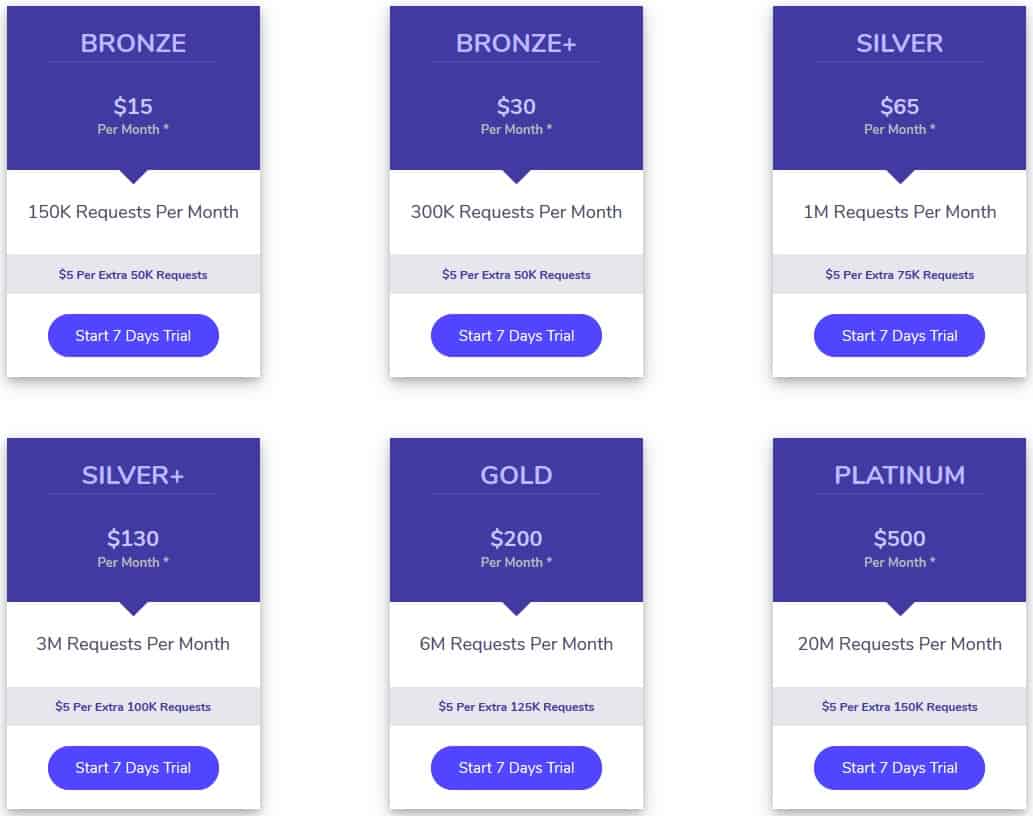
Monthly plans can all be upgraded to annual plans for even more benefits, and every plan has a 7-day free trial (limited to 100,000 requests) to check out firsthand what you’re getting into and how it works.
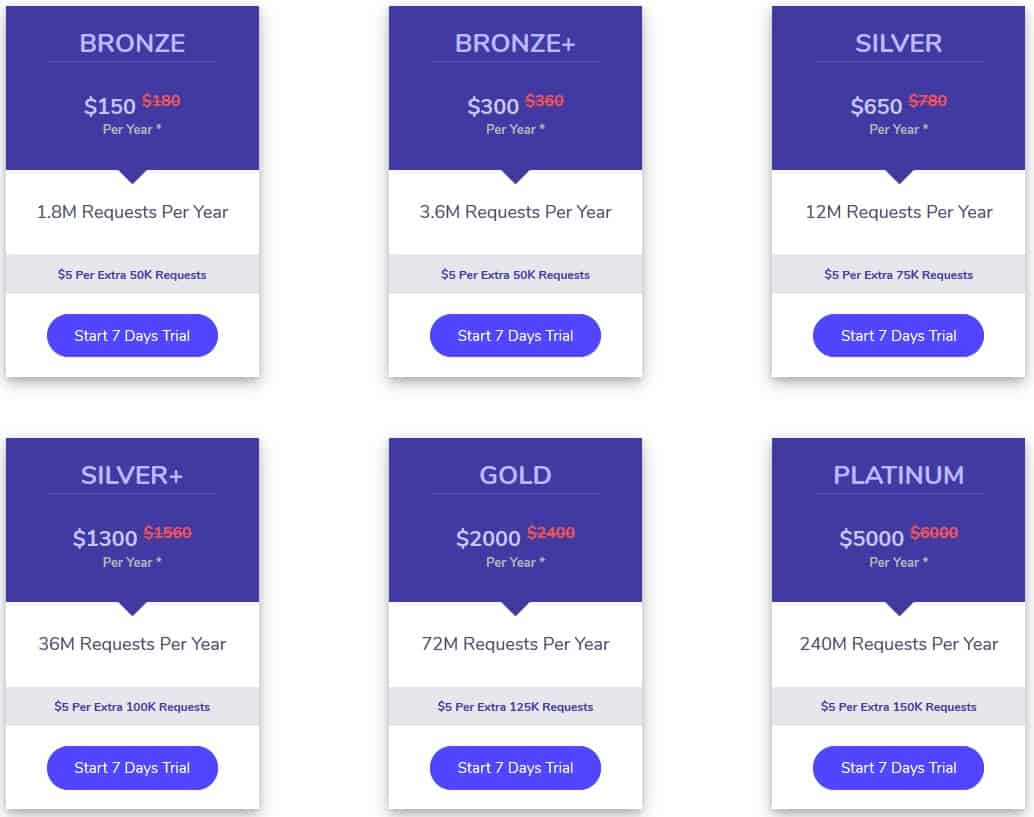
Summary
On first glance making requests for geolocation in PHP can seem like taxing work, but when you get acquainted with the call method and main parameters everything else falls into place quickly. Add that to the great service you’ll be getting with IP Geolocation and you’ll find yourself making geolocation requests by the thousands in no time.